Using SLF4J With Apache Log4j: An Example
June 7, 2019 • Java • 3 minutes to read • Edit
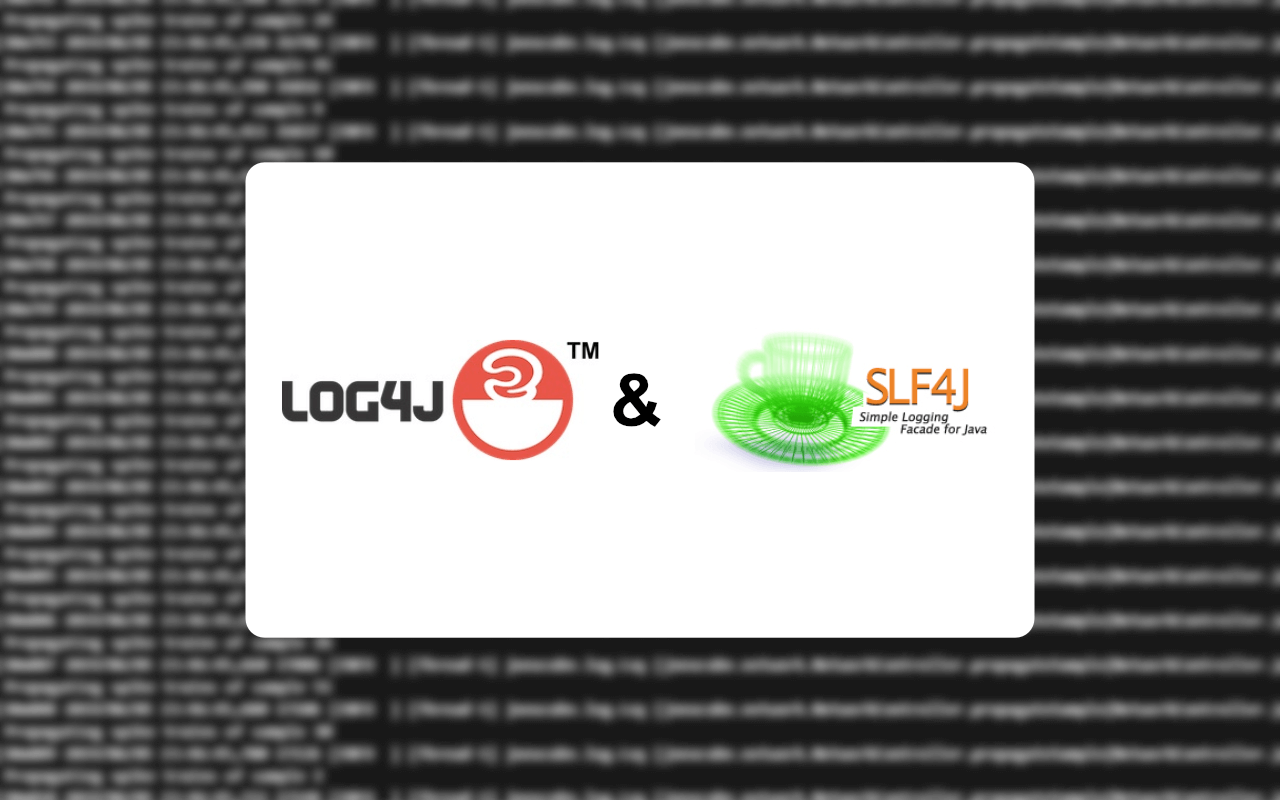
Logging is essential when developing a software to keep a track on what is happening internally. This blog will show you how to use SLF4J with Apache Log4j.
1. Requirements
This example assumes that you are familiar using Maven dependency manager. All the dependencies are listed in pom.xml
2. What is logging?
In general, logging refers to the recording of activity. In Java, logging can be divided into three levels:
Logging
|
.------------+-------------.
| | |
Logger Formatter Handler
(Appender)
2.1. Logger
Logger is an object, which is called when you want to log a message. Logger has two main objects in it:
- Name
- Logging levels
2.1.1. Name
It is the class name of the application. For example com.gollahalli.UsingLog4JSLF4J
. You can use this by calling
logger.getName();
2.1.2. Levels
They are the logging events that can be called while running a program.
Log4J and SLF4J provides the following levels of events:
Log4J | SLF4J |
---|---|
ALL | - |
DEBUG | DEBUG |
ERROR | ERROR |
FATAL | - |
INFO | INFO |
OFF | - |
TRACE | TRACE |
WARN | WARN |
CATCHING | - |
2.2. Pattern Layout
For system output and file output, the pattern is the same. See log4j2.xml#L6
These are used to format the way the logs are displayed on the Terminal/Command Prompt or in the log files.
2.3. Appenders
In this example, there are three types of appenders
- Console
- File with Pattern (Async)
- JSON File (Async)
2.3.1. Console Appender
This type of error writes the log event to the console using either of the two output methods
System.out
System.err
It defaults to System.err
See log4j2.xml#L4-L7
2.3.2. File with Pattern
File
appenders are part of AsyncAppender
, that means the file is written on a different thread. A simple file appender can have the same pattern as the console pattern but instead of showing it on the console its written to the file.
See log4j2.xml#L9-L14 - for file logging
See log4j2.xml#L21-L24 - for Async
appender
2.3.3. JSON File (Async)
Using additional libraries such as, jackson
and jackson-binding
, log events can be exported to JSON objects. Using JsonLayout
under File
, the file written is a JSON file.
See log4j2.xml#L16-L19) - for JSON file logging
See log4j2.xml#L21-L24 - for Async
appender
3. What is Log4J?
Log4J is a logging library for Java developed by Apache Logging project, which is a part of Apache Software Foundation Project.
3.1. Why use Log4J when Java has java.util.logging?
Apache can do everything that java.util.logging
and more. Log4J can be used to log at the runtime. It also helps in lowering the performance cost. For more information see Apache Logging.
4. What is SLF4J?
Simple Logging Facade for Java (SLF4J) acts as an abstract layer for different types of logging libraries; this includes Log4J.
4.1. Why use SLF4J when Log4J has different types of log levels?
That’s correct, but what if you don’t want to use Log4J? And the person you give your code to has a different type of logger with them, that’s when SLF4J comes in handy. For more information see SLF4J.
5. All about log4j2.xml
There are three ways to configure Log4J. Using XML
, JSON
and properties
file. For the purpose of this tutorial, we will stick to XML
.
Overview of Configuration File
See log4j2.xml
<?xml version="1.0" encoding="UTF-8"?>
<Configuration ...>
<Appenders>
<!-- This is where your appenders go -->
</Appenders>
<Loggers>
<Root ...>
<!-- Refer to an appender from here -->
</Root>
</Loggers>
</Configuration>
For more information see Log4J Configuration.